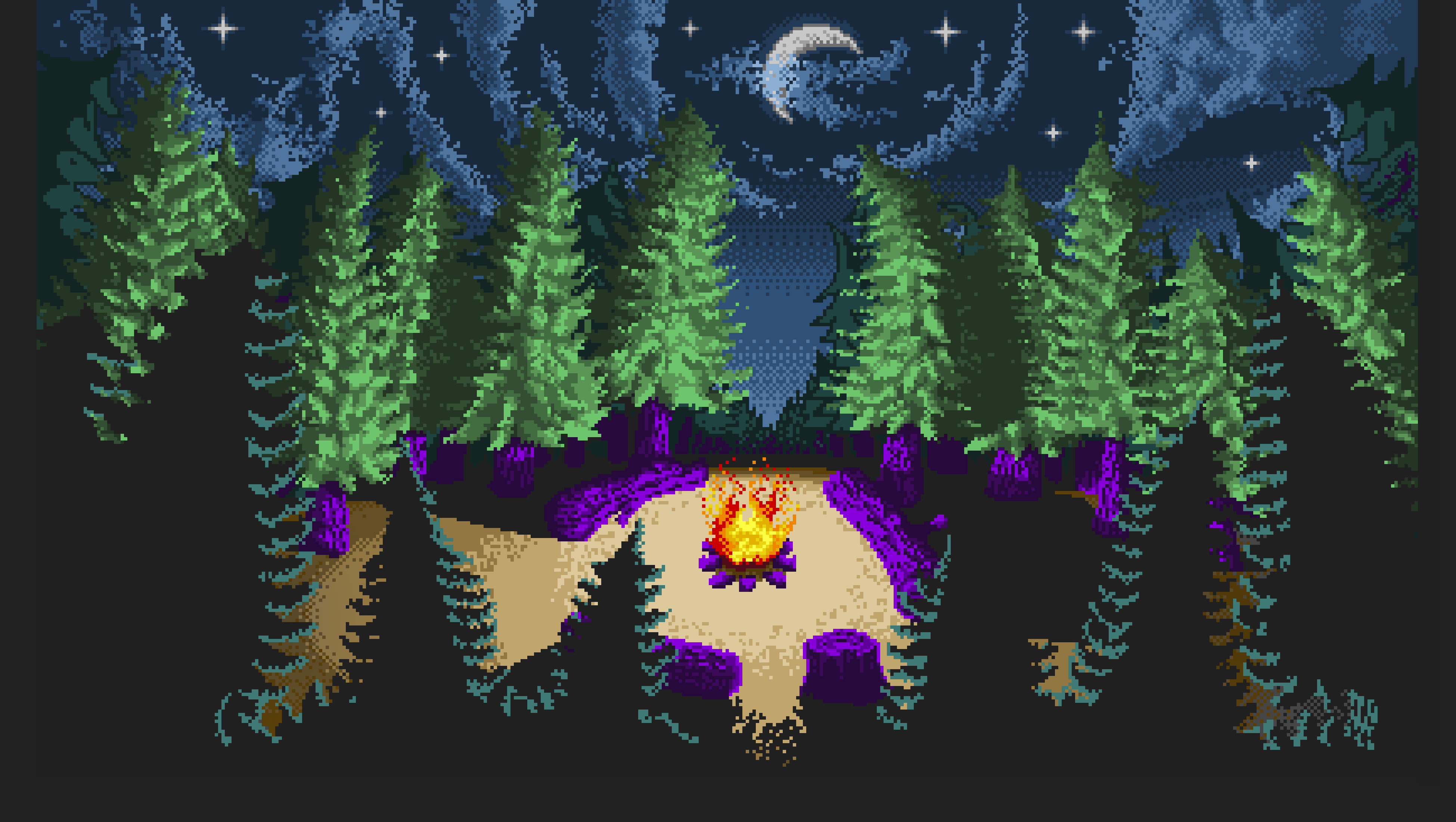
Setting Up Your Environment
The first step in our journey is to set up the environment by installing the necessary libraries. These libraries include Gradio for the web interface, transformers
for accessing pre-trained models, sentencepiece
for text processing, and accelerate
for optimizing the computation. Run the following commands in your terminal or Jupyter notebook:
!pip install gradio transformers sentencepiece accelerate
Detailed Explanation:
- Gradio: A Python library to create customizable UIs for machine learning models.
- Transformers: Provides thousands of pre-trained models to perform tasks on texts.
- SentencePiece: A library for unsupervised text tokenization, crucial for processing text data for machine learning models.
- Accelerate: A library to accelerate computation on GPUs.
Writing Your First Lines of Code
After installing the required libraries, the next step is to import them into your Python script or notebook. This is how you do it:
import torch
from transformers import pipeline
import gradio as gr
torch
: The PyTorch library, used here for its powerful tensor operations and GPU acceleration.from transformers import pipeline
: Imports thepipeline
function from thetransformers
library, which simplifies the process of loading and using pre-trained models.import gradio as gr
: Imports Gradio, which we will use to create an interactive web interface for our model.
Exploring the Code
Now, let’s load the Dolly model using the pipeline
function from the transformers
library. This will allow us to use the model for generating text based on the input we provide:
dolly_pipeline = pipeline(model="databricks/dolly-v2-3b",
torch_dtype=torch.bfloat16,
trust_remote_code=True,
device_map="auto")
model="databricks/dolly-v2-3b"
: Specifies the Dolly model we are using.torch_dtype=torch.bfloat16
: Sets the data type to Brain Floating Point Format (bfloat16) to reduce memory usage and speed up computation without significantly affecting the accuracy.trust_remote_code=True
: Allows the loading of custom operations from the model. This is important for complex models like Dolly.device_map="auto"
: Automatically selects the best available device (CPU or GPU) for model inference, optimizing performance.
Creating an Interactive Function
With the model loaded, we will now define a function that takes user input, generates a context, and uses the Dolly model to provide a response. Here’s how you can define this function:
def get_completion_dolly(input):
system = """
You are an expert Physicist.
You are good at explaining Physics concepts in simple words.
Help as much as you can.
"""
prompt = f"#### System: {system}\n#### User: \n{input}\n\n#### Response from Dolly-v2-3b:"
print(prompt)
dolly_response = dolly_pipeline(prompt, max_new_tokens=500)
return dolly_response[0]["generated_text"]
- The function
get_completion_dolly
takes a stringinput
as its argument. - Inside the function, a context
system
is defined, positioning the AI as an expert physicist. - The
prompt
combines this context with the user’s input, formatting it as a conversation. dolly_pipeline(prompt, max_new_tokens=500)
sends this prompt to the Dolly model and generates a response, limited to 500 tokens.- The function returns the text generated by the model, providing a coherent and context-aware answer.
Deploying the Gradio Interface
The final step is to create a Gradio interface that users can interact with. This interface will use the get_completion_dolly
function to generate answers to user questions:
iface = gr.Interface(fn=get_completion_dolly,
inputs=[gr.Textbox(label="Insert Prompt Here", lines=6)],
outputs=[gr.Textbox(label="Your Answer Here", lines=3)],
title="My AI Physics Teacher",
examples=["Explain the difference between nuclear fusion and fission.",
"Why is the Sky blue?"])
iface.launch(share=True)
gr.Interface()
creates a new Gradio interface. Thefn
parameter is set to our functionget_completion_dolly
, which means this function will be called when the user submits input.inputs=[gr.Textbox(...)]
defines the input component of the interface. Here, it’s a textbox where users can type their questions.outputs=[gr.Textbox(...)]
specifies how the output (the model’s response) will be displayed, which is also in a textbox.title="My AI Physics Teacher"
sets the title of the web interface.examples=[...]
provides example questions to help users understand what kind of queries they can ask.iface.launch(share=True)
starts the Gradio app and makes it accessible via a public URL so that anyone can use the AI model.
Learn How To Build AI Projects
Learn How To Build AI Projects
Now, if you are interested in upskilling in 2024 with AI development, check out this 6 AI advanced projects with Golang where you will learn about building with AI and getting the best knowledge there is currently. Here’s the link.
Conclusion
Congratulations! You’ve successfully built an interactive AI application using Gradio and the Dolly model. This guide provided a step-by-step walkthrough, from setting up the environment to deploying the web interface, to help you understand and create your interactive AI project.